I'm working on DandeGUI, a Common Lisp GUI library for simple text and graphics output on Medley Interlisp. The name, pronounced “dandy guy”, is a nod to the Dandelion workstation, one of the Xerox D-machines Interlisp-D ran on in the 1980s.
DandeGUI allows the creation and management of windows for stream-based text and graphics output. It captures typical GUI patterns of the Medley environment such as printing text to a window instead of the standard output. The main window of this screenshot was created by the code shown above it.
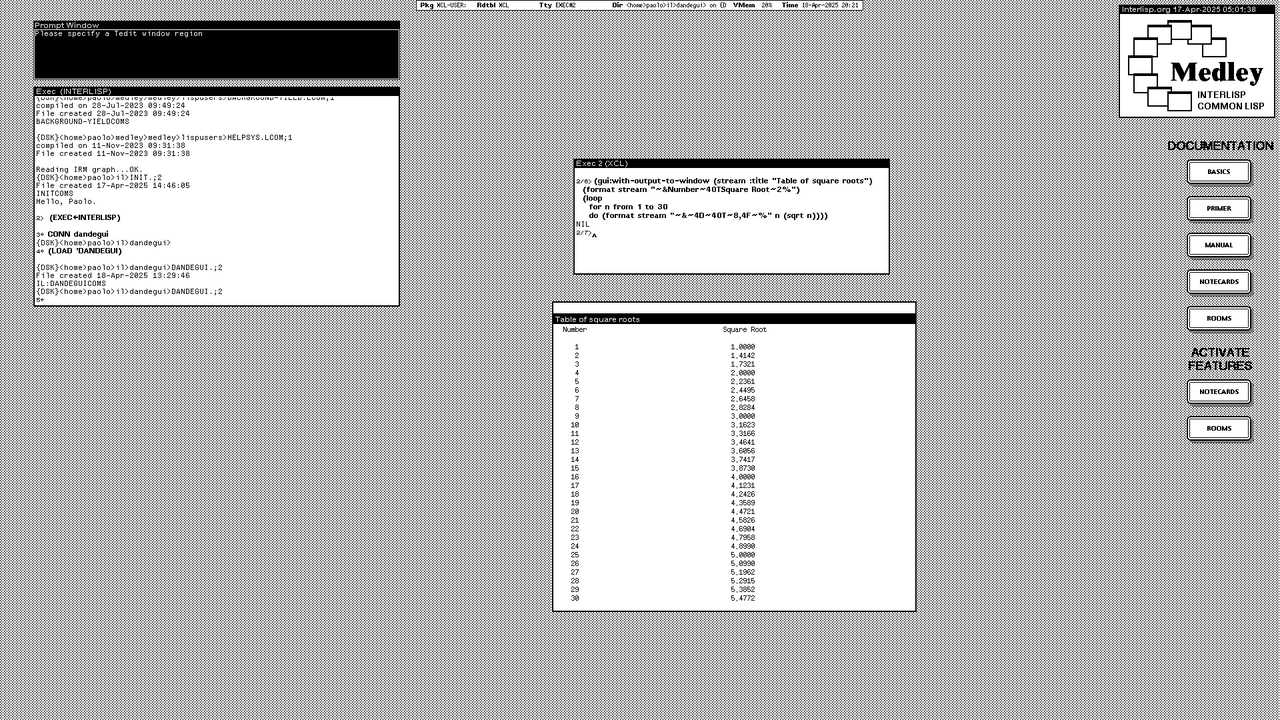
The library is written in Common Lisp and exposes its functionality as an API callable from Common Lisp and Interlisp code.
Motivations
In most of my prior Lisp projects I wrote programs that print text to windows.
In general these windows are actually not bare Medley windows but running instances of the TEdit rich-text editor. Driving a full editor instead of directly creating windows may be overkill, but I get for free content scrolling as well as window resizing and repainting which TEdit handles automatically.
Moreover, TEdit windows have an associated TEXTSTREAM
, an Interlisp data structure for text stream I/O. A TEXTSTREAM
can be passed to any Common Lisp or Interlisp output function that takes a stream as an argument such as PRINC
, FORMAT
, and PRIN1
. For example, if S
is the TEXTSTREAM
associated with a TEdit window, (FORMAT S "~&Hello, Medley!~%")
inserts the text “Hello, Medley!” in the window at the position of the cursor. Simple and versatile.
As I wrote more GUI code, recurring patterns and boilerplate emerged. These programs usually create a new TEdit window; set up the title and other options; fetch the associated text stream; and return it for further use. The rest of the program prints application specific text to the stream and hence to the window.
These patterns were ripe for abstracting and packaging in a library that other programs can call. This work is also good experience with API design.
Usage
An example best illustrates what DandeGUI can do and how to use it. Suppose you want to display in a window some text such as a table of square roots. This code creates the table in the screenshot above:
(gui:with-output-to-window (stream :title "Table of square roots")
(format stream "~&Number~40TSquare Root~2%")
(loop
for n from 1 to 30
do (format stream "~&~4D~40T~8,4F~%" n (sqrt n))))
DandeGUI exports all the public symbols from the DANDEGUI
package with nickname GUI
. The macro GUI:WITH-OUTPUT-TO-WINDOW
creates a new TEdit window with title specified by :TITLE
, and establishes a context in which the variable STREAM
is bound to the stream associated with the window. The rest of the code prints the table by repeatedly calling the Common Lisp function FORMAT
with the stream.
GUI:WITH-OUTPUT-TO-WINDOW
is best suited for one-off output as the stream is no longer accessible outside of its scope.
To retain the stream and send output in a series of steps, or from different parts of the program, you need a combination of GUI:OPEN-WINDOW-STREAM
and GUI:WITH-WINDOW-STREAM
. The former opens and returns a new window stream which may later be used by FORMAT
and other stream output functions. These functions must be wrapped in calls to the macro GUI:WITH-WINDOW-STREAM
to establish a context in which a variable is bound to the appropriate stream.
The DandeGUI documentation on the project repository provides more details, sample code, and the API reference.
Design
DandeGUI is a thin wrapper around the Interlisp system facilities that provide the underlying functionality.
The main reason for a thin wrapper is to have a simple API that covers the most common user interface patterns. Despite the simplicity, the library takes care of a lot of the complexity of managing Medley GUIs such as content scrolling and window repainting and resizing.
A thin wrapper doesn't hide much the data structures ubiquitous in Medley GUIs such as menus and font descriptors. This is a plus as the programmer leverages prior knowledge of these facilities.
So far I have no clear idea how DandeGUI may evolve. One more reason not to deepen the wrapper too much without a clear direction.
The user needs not know whether DandeGUI packs TEdit or ordinary windows under the hood. Therefore, another design goal is to hide this implementation detail. DandeGUI, for example, disables the main command menu of TEdit and sets the editor buffer to read-only so that typing in the window doesn't change the text accidentally.
Using Medley Common Lisp
DandeGUI relies on basic Common Lisp features. Although the Medley Common Lisp implementation is not ANSI compliant it provides all I need, with one exception.
The function DANDEGUI:WINDOW-TITLE
returns the title of a window and allows to set it with a SETF
function. However, the SEdit structure editor and the File Manager of Medley don't support or track function names that are lists such as (SETF WINDOW-TITLE)
. A good workaround is to define SETF
functions with DEFSETF
which Medley does support along with the CLtL macro DEFINE-SETF-METHOD
.
Next steps
At present DandeGUI doesn't do much more than what described here.
To enhance this foundation I'll likely allow to clear existing text and give control over where to insert text in windows, such as at the beginning or end. DandeGUI will also have rich text facilities like printing in bold or changing fonts.
The windows of some of my programs have an attached menu of commands and a status area for displaying errors and other messages. I will eventually implement such menu-ed windows.
To support programs that do graphics output I plan to leverage the functionality of Sketch for graphics in a way similar to how I build upon TEdit for text.
Sketch is the line drawing editor of Medley. The Interlisp graphics primitives require as an argument a DISPLAYSTREAM
, a data stracture that represents an output sink for graphics. It is possible to use the Sketch drawing area as an output destination by associating a DISPLAYSTREAM
with the editor's window. Like TEdit, Sketch takes care of repainting content as well as window scrolling and resizing. In other words, DISPLAYSTREAM
is to Sketch what TEXTSTREAM
is to TEdit.
DandeGUI will create and manage Sketch windows with associated streams suitable for use as the DISPLAYSTREAM
the graphics primitives require.
#DandeGUI #CommonLisp #Interlisp #Lisp
Discuss...
Email | Reply @amoroso@oldbytes.space